C#でデスクトップ上に四角枠を表示するコードを記載します。
↓の青枠みたいに表示します。
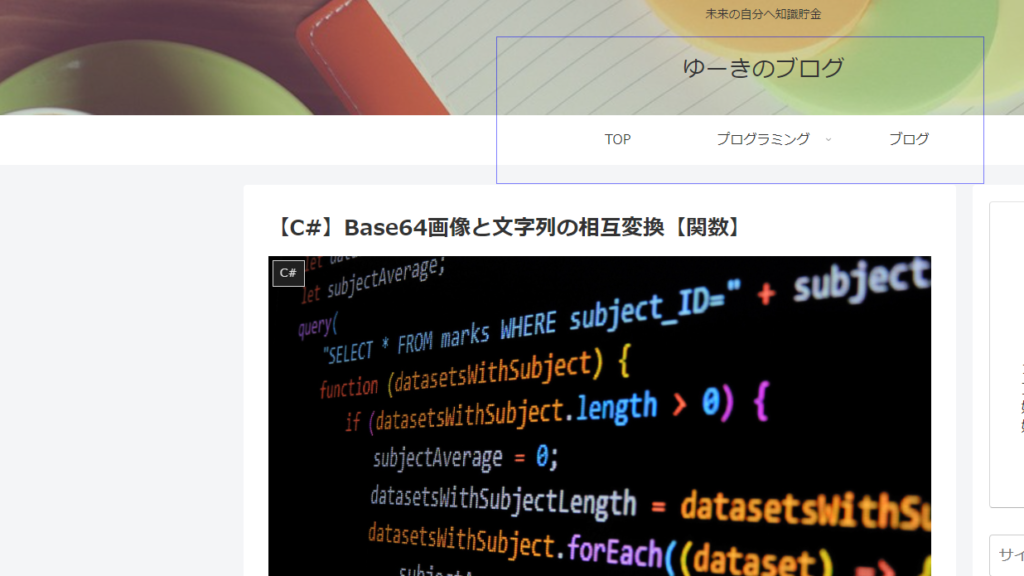
■DialogRegionView.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace SCSupportTool
{
public partial class DialogRegionView : Form
{
//マウスのクリック位置を記憶
private Point mousePoint;
public DialogRegionView()
{
InitializeComponent();
}
private void DialogRegionView_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Escape)
{
this.DialogResult = DialogResult.Cancel;
this.Close();
}
}
private void pictureBox1_MouseDown(object sender, MouseEventArgs e)
{
// 画面を掴んで移動できるように
if ((e.Button & MouseButtons.Left) == MouseButtons.Left)
{
//位置を記憶する
mousePoint = new Point(e.X, e.Y);
}
}
private void pictureBox1_MouseUp(object sender, MouseEventArgs e)
{
}
private void pictureBox1_MouseMove(object sender, MouseEventArgs e)
{
// 画面を掴んで移動できるように
if ((e.Button & MouseButtons.Left) == MouseButtons.Left)
{
this.Left += e.X - mousePoint.X;
this.Top += e.Y - mousePoint.Y;
}
}
public void SetRegion(Rectangle region)
{
this.Location = region.Location;
this.Size = region.Size;
}
}
}
■DialogRegionView.Designer.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace SCSupportTool
{
public partial class DialogRegionView : Form
{
//マウスのクリック位置を記憶
private Point mousePoint;
public DialogRegionView()
{
InitializeComponent();
}
private void DialogRegionView_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.Escape)
{
this.DialogResult = DialogResult.Cancel;
this.Close();
}
}
private void pictureBox1_MouseDown(object sender, MouseEventArgs e)
{
// 画面を掴んで移動できるように
if ((e.Button & MouseButtons.Left) == MouseButtons.Left)
{
//位置を記憶する
mousePoint = new Point(e.X, e.Y);
}
}
private void pictureBox1_MouseUp(object sender, MouseEventArgs e)
{
}
private void pictureBox1_MouseMove(object sender, MouseEventArgs e)
{
// 画面を掴んで移動できるように
if ((e.Button & MouseButtons.Left) == MouseButtons.Left)
{
this.Left += e.X - mousePoint.X;
this.Top += e.Y - mousePoint.Y;
}
}
public void SetRegion(Rectangle region)
{
this.Location = region.Location;
this.Size = region.Size;
}
}
}
■使い方
public enum eRegion
{
Sample1,
Sample2,
Sample3,
_Count,
}
private DialogRegionView[] dlgRegionViews = new DialogRegionView[(int)eRegion._Count];
private void ShowRegion(eRegion iRegion)
{
Rectangle region = Program.App.Param.Regions[(int)iRegion];
if (this.dlgRegionViews[(int)iRegion] == null)
{
this.dlgRegionViews[(int)iRegion] = new DialogRegionView();
DialogRegionView view = this.dlgRegionViews[(int)iRegion];
view.SetRegion(region);
view.Show();
}
}
private void InvisibleRegion(eRegion iRegion)
{
Rectangle region = Program.App.Param.Regions[(int)iRegion];
if (this.dlgRegionViews[(int)iRegion] != null)
{
this.dlgRegionViews[(int)iRegion].Close();
this.dlgRegionViews[(int)iRegion] = null;
}
以上!
コメント